Introduction to Python (Part 2)
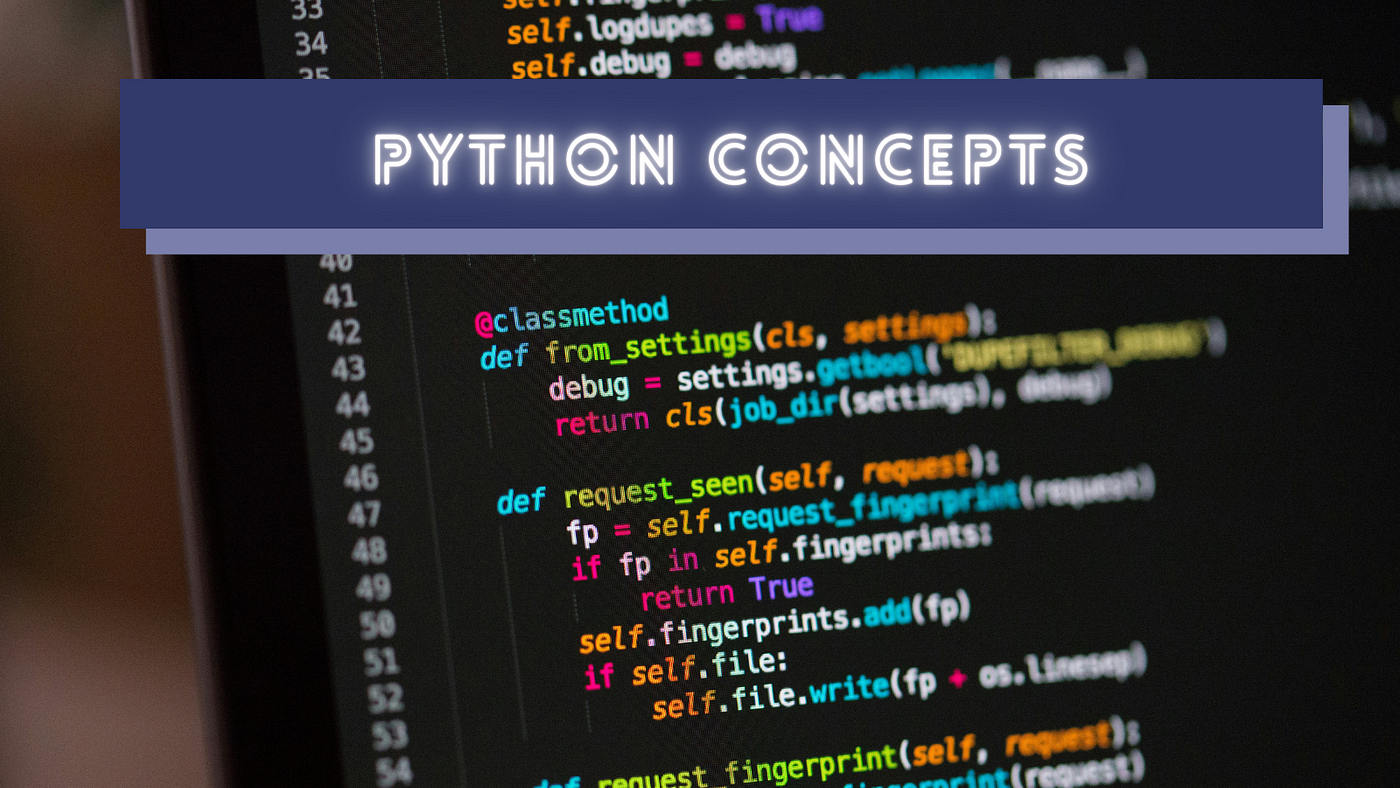
Recap
Welcome back to our Python course! Last time we covered the print() function, all the different types of data types, variables, and how to set up Visual Studio Code. All of this is very important, so if you haven’t checked out that article, make sure to do so! Now let’s jump in.
Comments
Comments are things that the computer does not read in the code. They can be used to organize your code, make it more readable, explain your code, and write down notes.
Oftentimes comments are useful for when you are sharing your code with other developers or those around you in a working environment.
Comments start with a # and the computer will ignore the rest of the line after that.
print(“Hello world”) # prints “Hello world”
The above code will only print “Hello world” and ignore everything after that!
Additionally, you can use comments to prevent code from running when you are finding errors in your code, or debugging.
#print(“Hello world”)
print(“I didn’t say anything!”)
The computer won’t print “Hello world” at all!
Operators
Python can be used to do math! There are 7 operators in Python:
- Addition: +
- Subtraction: –
- Multiplication: *
- Division: /
- Remainder: %
- Exponents: **
- Floor division: //
The most simple one is addition. Add two numbers together – or even two strings. (Remember when we did concatenation in the last article?)
Using print(2+2), you’ll see the output is 4!
The next one subtraction, works similarly. Using print(5-2), you’ll see the output is 3!
This also works for negative numbers! print(-3+2) returns -1, and print(-3–2) returns -1 too.
Multiplication and division work like they would in normal math. You see where this is going.
Now let’s talk about the three more special ones. The % returns the remainder when two numbers are divided. We know that 11 divided by 5 is 2 remainder 1. So, when we do print(11 % 2), sure enough, we get 1.
** is exponents. Let’s say we wanted to know what the cube of 3 is 27. print(3 ** 3) returns 27. Here’s a basic formula to help you: [number you want to raise] ** [number to raise it to the power of]
> Can you find 10 to the power of 32 using Python?
print(10 ** 32) is how you do it. (It’s 100000000000000000000000000000000 – that’s a big number!)
Type Conversion / Type Casting
Last article, we learned the different types of data types. One useful thing to learn in Python is how to convert between them.
- The int() function converts a string or a float into an integer.
Here’s some examples of how to use it:
x = int(5) -> x will be 5
y = int(3.14) -> y will be 3 (if it is 3.18, it will still be 3; it simply eliminates the decimal)
z = int(“5”) -> z will be 5
- The float() function converts a string or an integer into a float.
Here’s some examples of how to use it:
w = float(5) -> w will be 5.0
x = float(3.14) -> x will be 3.14
y = float(“5”) -> y will be 5.0
z = float(“3.14”) -> z will be 3.14
- The str() function converts a float or integer into a string.
Here’s some examples of how to use it:
x = str(“3”) -> x will be “3”
y = str(2) -> y will be “2”
z = str(“3.14”) -> z will be 3.14
Lists
In Python, lists are used to assign multiple items, separated by commas, to a single variable. It is one of the built-in ways to store data – called a collection or an array! Each type of collection has unique properties.
Lists have a certain order, can be changed, and allow duplicates.
Properties of Lists
The order of lists cannot be changed in the code. When you add an item to the list in the code, it goes to the end. This is sensible because:
They are indexed, which means each one has a number assigned to it. The first item of the list is [0], the second item of the list is [1], the third the list is [2].
So, for example, if I had a list called list1, I could find the first item using list[0].
Common Error: Get in the habit of thinking that the first item of the list is [0], not [1], which is the second item in the list.
This indexing allows multiple items to be the same in the list. (Or, duplicate values!)
However, lists are quite flexible in that items can be changed, added, or removed from the list.
A list can contain any of our data types.
Examples:
movies = [“Star Wars”, “Back to the Future”, “Alien”] # this is a simple example
testscores = [98, 97, 85, 72, 98] # the last one is a duplicate, but it still works!
list1 = [“abc”, 123, True, “def”, 456] # you can mix a bunch of different data types!
list2 = [movies, testscores, list1] # you can even put lists inside of lists (and
other data collections, too)!
Exercises in Interacting With Lists
> Can you print True by only using one of the above lists?
Yes! We know the 3rd item of list1 is True, so all we have to do is: print(list1[2])
> More advanced: can you print True by only using list2?
We already know that the 3rd item of list1 is True. In list2, list1 is the 3rd and last element. Therefore, list2[2] is list1. So all we have to do is: print(list2[2][2])
As mentioned before, lists can be changed in your code.
Adding Items to Lists
You can add list items in a number of ways:
- .append() is used to add an item at the end of your list.
color_list = [“red”, “blue”, “green]
color_list.append(“yellow”)
Now color_list = [“red”, “blue”, “green”, “yellow”]
- .insert() is used to insert an item to a specific position (or index) in your list.
color_list = [“red”, “blue”, “green”]
color_list.insert(1, “yellow”)
Now color_list = [“red”, “yellow”, “blue”, “green”]
Remember: Saying insert(1, “yellow”) is like saying “put yellow into the 2nd position in the list.” (Not the 1st!) It follows the same rule as indexes.
Removing Items From Lists
You can also remove list items in a number of ways:
- .remove() is used to remove a specific item from your list.
color_list = [“red”, “blue”, “green”, “yellow”]
color_list.remove(“yellow”)
Now color_list = [“red”, “blue”, “green”]
- .pop() is used to remove an item of a specific index from your list.
color_list = [“red”, “blue”, “green”, “yellow”]
color_list.pop(1)
Now color_list = [“red”, “green”, “yellow”]
If no number is given in .pop(), the last item of the list will be removed.
color_list = [“red”, “blue”, “green”, “yellow”]
color_list.pop()
Now color_list = [“red”, “blue”, “green”]
- .clear() is used to empty a list – it’s still there but has no items in it.
color_list = [“red”, “blue”, “green”, “yellow”]
color_list.clear()
Now color_list = [].
Summary
In this article, we reviewed comments, mathematical operators, converting data types, and lists. Lists are extremely useful and common in Python, so I recommend you review those well! In the next article, we’ll take a look at more data collections like dictionaries and how to interact with them, among other things. I hope you enjoyed this article. See you in the next one as we continue our Python journey!